CoffeeScript is a programming language built on JavaScript, which is compiled into efficient JavaScript so you can run it in a web browser or through technologies such as Node.js for server-side applications. Use it. The compilation process is usually simple, and the resulting JavaScript adheres to many best practices.
In fact, it just extracts the useful parts of JS and adds some syntactic sugar!
Unless you are very familiar with JS, the execution efficiency of COFFEE is probably higher than handwriting, and it is elegant.
But if you are really familiar with JS, the code you handwrite in JS should be the same as the code compiled by coffee.
So choosing COFFEE can save you a keyboard. .
Let’s take a look at the comparison code first (CoffeeScript on the left):
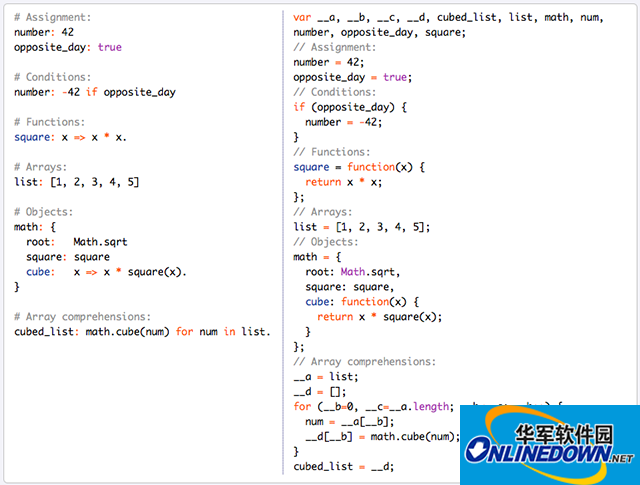
The appeal of CoffeeScript:
1. Provide a relatively simple syntax that reduces boilerplate code, such as brackets and commas;
2. Use spaces as a way to organize code blocks;
3. Provide a simple syntax for expressing functions;
4. Provide class-based inheritance (optional, but very useful when developing applications)
Prerequisites:
CoffeeScript uses Nodejs's package manager and is distributed as a package of Node.js.
CoffeeScript must be compiled, and its compiler is actually written using CoffeeScript, so a JavaScript runtime is required to complete its compilation.
Installation method:
sudo npm install -g coffee-script
After successful installation, we should be able to find the coffee command on the command line. coffee -v What I see here is 1.6.0. Using the coffee command, .coffee files can be compiled into js files. Using the coffeescript format to write javascript is said to save two-thirds of the code.
Basic usage:
-c,--compile Compile .coffee into .js file
-w,--watch Monitor file changes and output monitoring results
-o,--output [DIR] Output the compiled result file to the specified directory
-p,--print will compile the results
-l,--lint If jsl (javascript lint) is installed, use lint to check the code
-s,--stdio Use the output of other programs as the standard input of coffee and obtain the standard output of JavaScript.
-e,--eval command line form
Install:
Have you ever wished you could run JavaScript from the command line? Not that I have, but CoffeeScript might change that. With Node.js, you can run JavaScript from the command line or as part of an execution script. This primary feature of Node.js allows the execution of CoffeeScript code on the command line, which provides the runtime required by the CoffeeScript compiler (written in CoffeeScript).
The first step is to install Node.js. There are several installation options; you can compile the source code or run one of the installers available for various systems. Run node -v from the command line to confirm that Node.js is installed and in the path.
Node.js gives you an added bonus: the Node Package Manager (NPM). After running npm -v from the command line to confirm that NPM has been installed and is in the path, you can follow the steps below to install CoffeeScript using NPM.
1. Run npm install --global coffee-script through the command line.
The --global flag allows CoffeeScript to be used system-wide rather than just for a specific project.
2. The npm command should output information such as /usr/bin/coffee -> /usr/lib/node_modules/coffee-script/bin/coffee.
NPM creates a shortcut in /usr/bin, so now the coffee executable is in the correct path, which is the CoffeeScript compiler and interpreter.
3. To verify that the coffee executable file is in the path, run coffee -v from the command line.
There is one last step left to make sure that the CoffeeScript environment is set up correctly. In order to make CoffeeScript available to any started Node.js process, you need to add it to what Node.js calls NODE_PATH. When encountering an unrecognized function, Node.js will retrieve it by searching NODE_PATH. Some modules (libraries).
For the examples in this article, Node.js is used primarily as the runtime for the CoffeeScript executable. The easiest thing to do is to simply add all NPM modules to NODE_PATH. To find out where the NPM modules are located, type npm ls -g. You will need to add an environment variable that points NODE_PATH to this location. For example, if the output of npm ls -g is /usr/lib, the module is located in the /usr/lib/node_modules directory. To set a NODE_PATH environment variable, run export NODE_PATH=/usr/lib/node_modules.
You can simplify things further by putting the above commands into a startup script (say ~/.bash_profile). To verify the changes, execute Node to start a Node.js shell and enter require('coffee-script'). The Node.js shell should load the CoffeeScript library. If there is no problem with execution, the CoffeeScript environment is ready to use. Now you can start your journey of exploring CoffeeScript starting with the compiler.
compiler
Running the CoffeeScript compiler is as easy as typing coffee -c, which starts CoffeeScript's read-evaluate-print-loop (REPL). To run the compiler, you need to pass it a CoffeScript file that you want to compile. Create a file called cup0.coffee and paste the contents of Listing 2 into the file.
Listing 2. Cup 0
for i in [0..5]
console.log "Hello #{i}"
You might be wondering what these two lines of code in Listing 2 do. Listing 3 shows the output of running coffee cup0.coffee.
Listing 3. Running the first CoffeeScript
$ coffee cup0.coffee
Hello 0
Hello 1
Hello 2
Hello 3
Hello 4
Hello 5
To get a clearer idea of what's going on, try running the compiler and typing coffee -c cup0.coffee. This command creates a file called cup0.js. Listing 4 shows the contents of cup0.js. .
CoffeeScript 1.9.3 is released. This version mainly has the following updates:
Bugfix for interpolation in the first key of an object literal in an implicit call.
Fixed broken error messages in the REPL, as well as a few minor bugs with the REPL.
Fixed source mappings for tokens at the beginning of lines when compiling with the --bareoption. This has the nice side effect of generating smaller source maPS.
Slight formatting improvement of compiled block comments.
Better error messages for on, off, yes and no.